Using Custom Metrics and Events
The Campaign player plugin includes a client-side API for attaching custom events and metrics to view events. The following example outlines how to track custom events and metrics.
- Obtain a reference to the Brightcove Player. This example assumes that an
id
ofmyPlayerID
has been added to the player embed code.var player = videojs(‘myPlayerID’);
- Listen for the Audience ready event,
audience:ready
.player.on(‘audience:ready’, function() { // player.audience is now available for use });
- To track a string value, call
player.audience.track(‘event[1-3]’, ‘value’)
. To track a numeric value, callplayer.audience.track(‘metric[1-3]’, value)
. The return value is a boolean indicating whether or not the event was sent successfully.// Store the string value “foo” in the slot event1 player.audience.track(‘event1’, ‘foo’); // Store the string value “bar” in the slot event2 player.audience.track(‘event2’, ‘bar’); // Store the decimal value 15.08 in slot metric1 player.audience.track(‘metric1’, 15.08); // Replace the value in slot metric1 with the number 10, // and validate that the call was sent successfully var result = player.audience.track(‘metric1’, 10); if (result) { console.log(‘metric sent successfully’); }
Tracking custom metadata example
The following code snippet uses custom metrics & events to track an event when a viewer enters fullscreen mode. At this event, we will also track metrics for the video’s current time (in seconds) and a custom field on the video called platform.
This example uses the Player API to:
- Track when the player enters full screen mode and track to
event1
- At the same time upon entering fullscreen mode, track the time the event occurs into
metric1
- Track a custom metadata field associated with the video into
metric2
<script>
// obtain a reference to your player
var player = videojs('myPlayer');
var audienceTrackingReady = false;
// listen when the audience plugin is ready
player.on('audience:ready', function() {
// player.audience is now available for use
audienceTrackingReady = true;
});
// track when viewer enters fullscreen mode
player.on('fullscreenchange', () => {
if (player.isFullscreen() & audienceTrackingReady) {
// The player is full screen, dispatch custom tracking
player.audience.track('event1', 'enteredfullscreen');
player.audience.track('metric1', player.currentTime());
player.audience.track('metric2', player.catalog.data.custom_fields['platform']);
}
});
</script>
Synchronization with marketing automation platforms
Custom events/metrics stored on a view event will sync for Marketo REST and Eloqua if the fields are defined on the custom activity or CDO. Campaign will also sync custom events/metrics to HubSpot if they're defined and to Salesforce if the integration is using a Mapping Type of Advanced and the events/metrics are mapped to something.
Marketo REST
For Marketo REST integrations, publishers should verify that the Brightcove Video View custom activity in Marketo has the custom event and metric fields. Custom activities are located under Admin > Database Management.
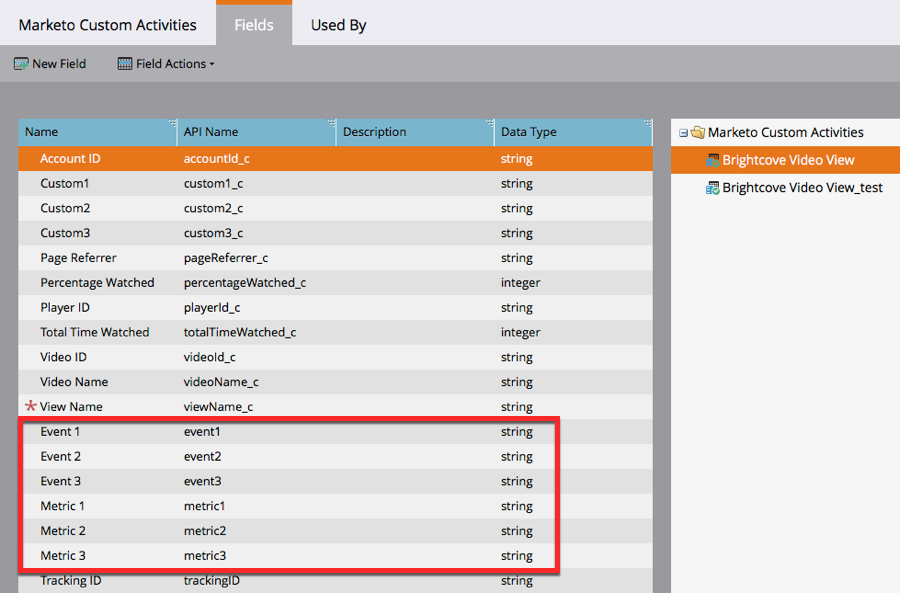
If the fields are not in the Brightcove Video view object:
- Update the Brightcove Video View custom activity to add the fields, or
- Contact Marketo Support and ask them to add the fields.
- After the fields have been added, return to Campaign and press the Complete Update button so Campaign can access the newly added fields.
Oracle Eloqua
For Eloqua integrations, publishers should verify that the BrightcoveVideoView custom object has the custom event and metric fields.
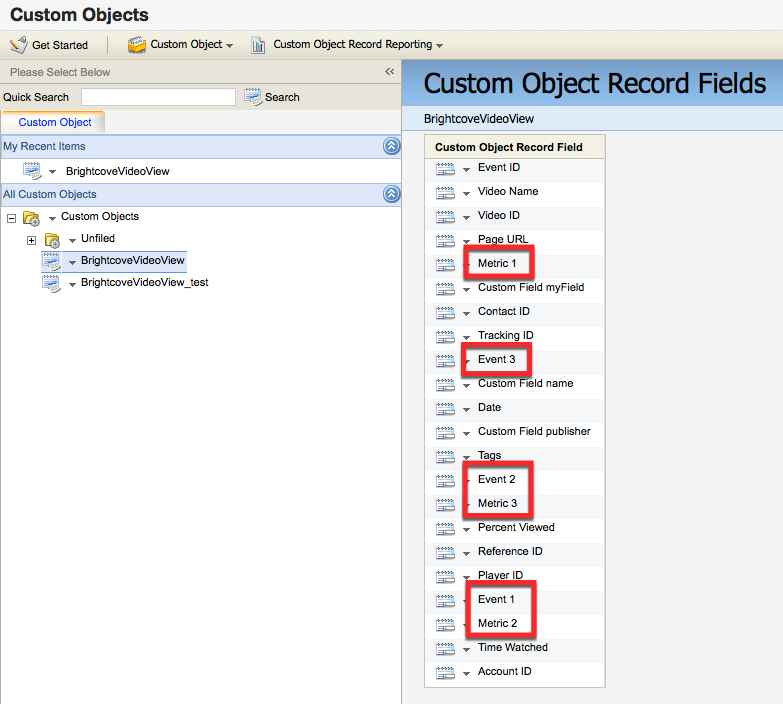
If the fields do not exist, the fields need to be added to the BrightcoveVideoView object by either:
- Reconnecting your Eloqua account, or
- Using the data mapping upgrade button.
Salesforce
For Salesforce, your Campaign connection should be using a Mapping Type of Advanced. The Event and Metric fields can be mapped to Salesforce objects.
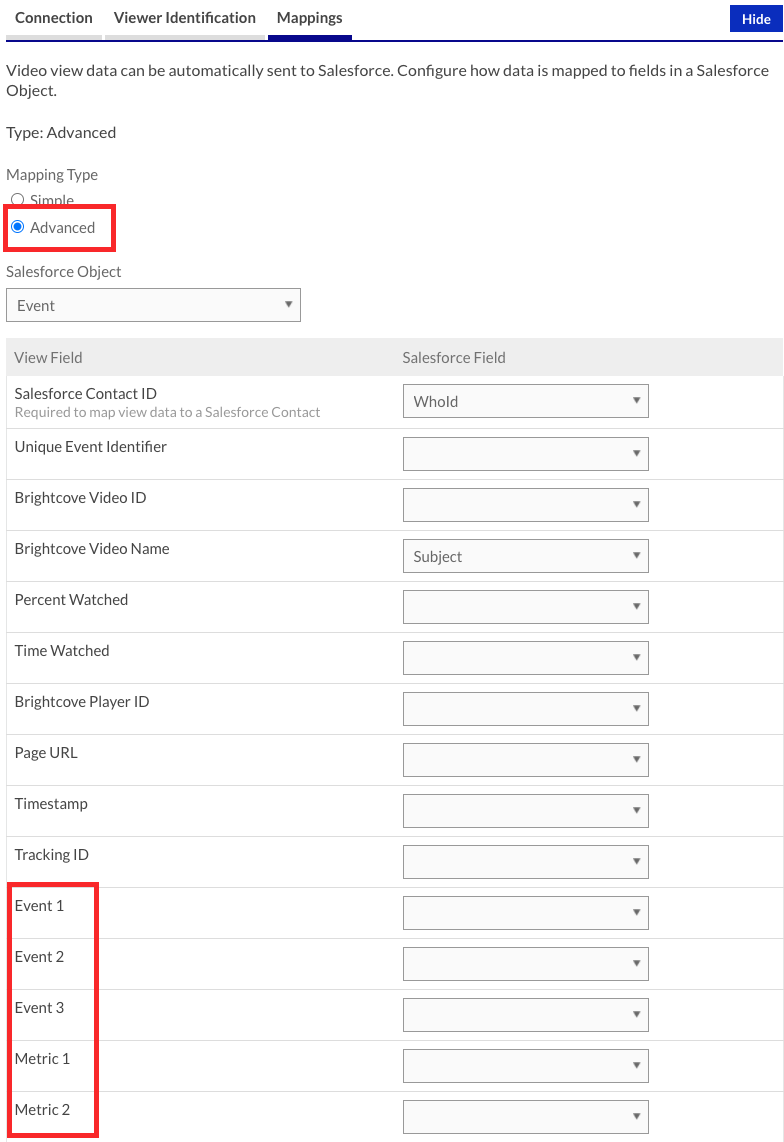
Notes
- The limit on string length for event slot values is 250 characters.
- If multiple values are sent to the same event or metric slot during a single viewing session, the existing value in the slot is replaced with the new value. In the following example, the final value stored in event1 is “click3”.
player.audience.track(‘event1’, ‘click1’); player.audience.track(‘event1’, ‘click2’); player.audience.track(‘event1’, ‘click3’);
- If a view event for the session does not exist yet then one will be created.
- If the result of
track()
is false indicating that the event failed to send, you can enable the verbose option on the Campaign plugin to see console logging with a specific reason why.
Viewing events and metrics
Custom events and metrics will appear in the Campaign UI. In the left navigation, click All Recent Activity. If any custom events or metrics have been recorded, the Show Interaction Events link will be enabled. Click the link to display the event/metric data.
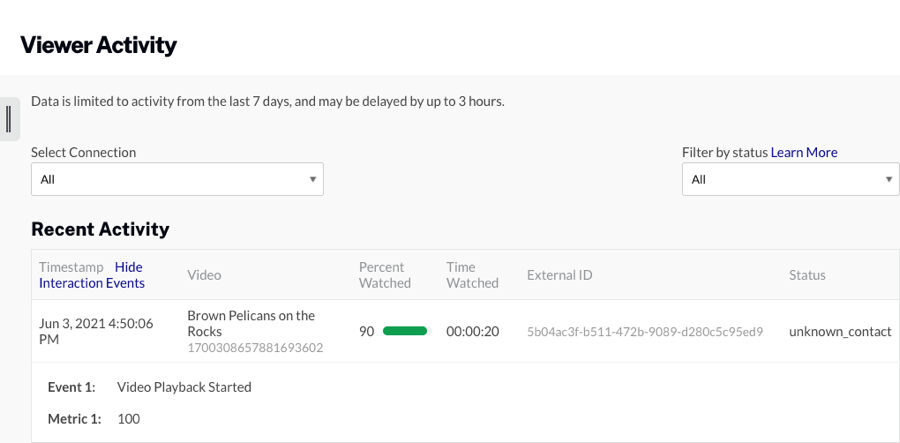
Enabling verbose logging
Verbose logging can be enabled by adding bcverbose=1
to the URL of the page with the player or by configuring the Campaign plugin with the "verbose": true
option:
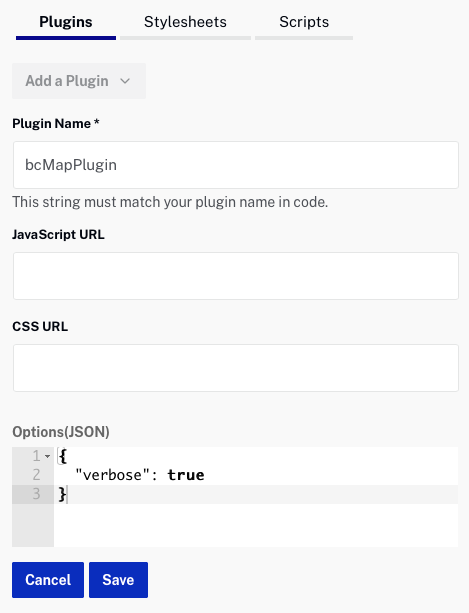